说明
本脚本是利用微信的公众平台进行信息推送的发送报警信息的.
可以通过这个链接 微信平台 了解, 可以注册团队,就没有时间限制了.
代码文件
- 需要安装requests模块,当初嫌麻烦,就直接使用的
requests
.- 脚本中最后,需要修改成实际的信息.
|
|
测试
907310009
为你添加用户时指定的帐号.1# python weixin.py 907310009 "你好,测试微信报警"
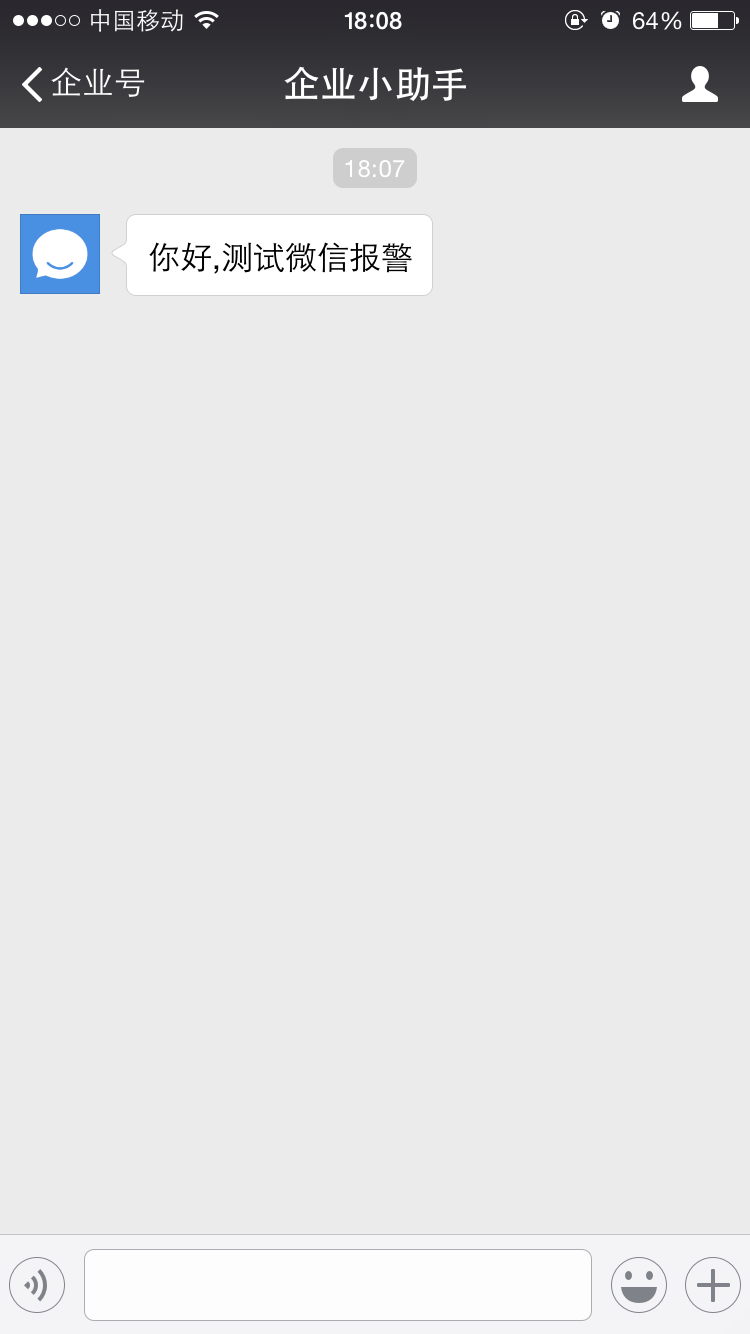
怎样查看agentid 和 Secret 等信息
下面用图说明吧,太详细的在后台自己可以琢磨琢磨
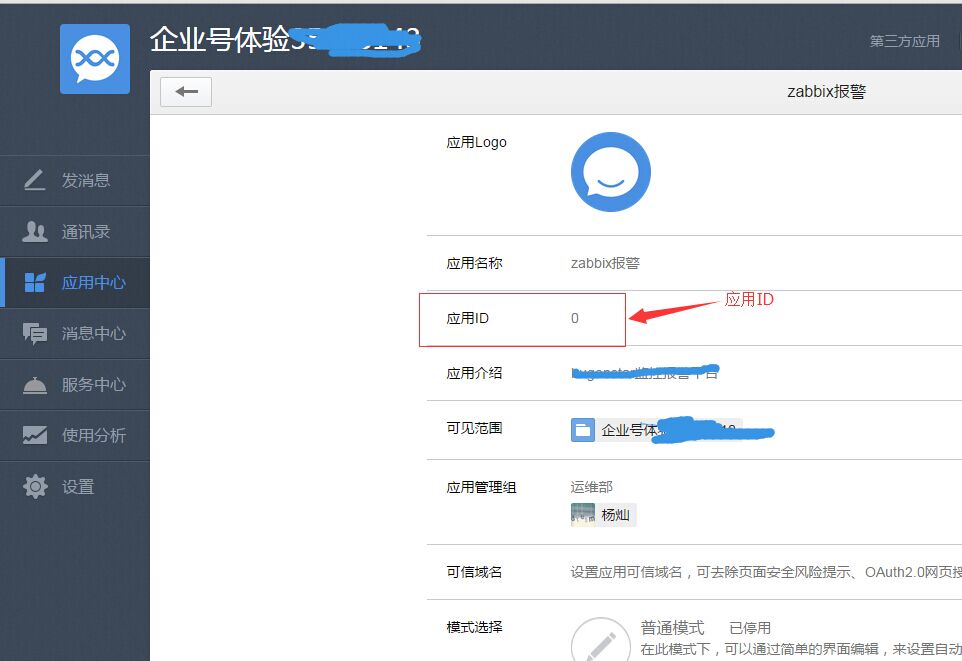
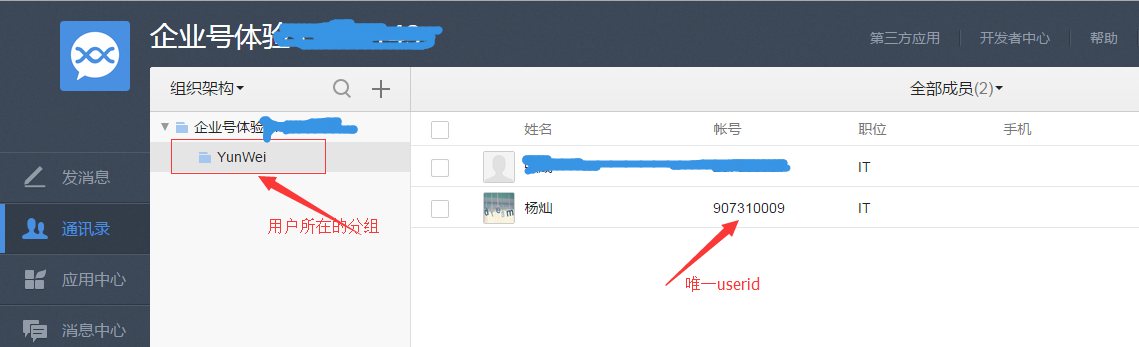
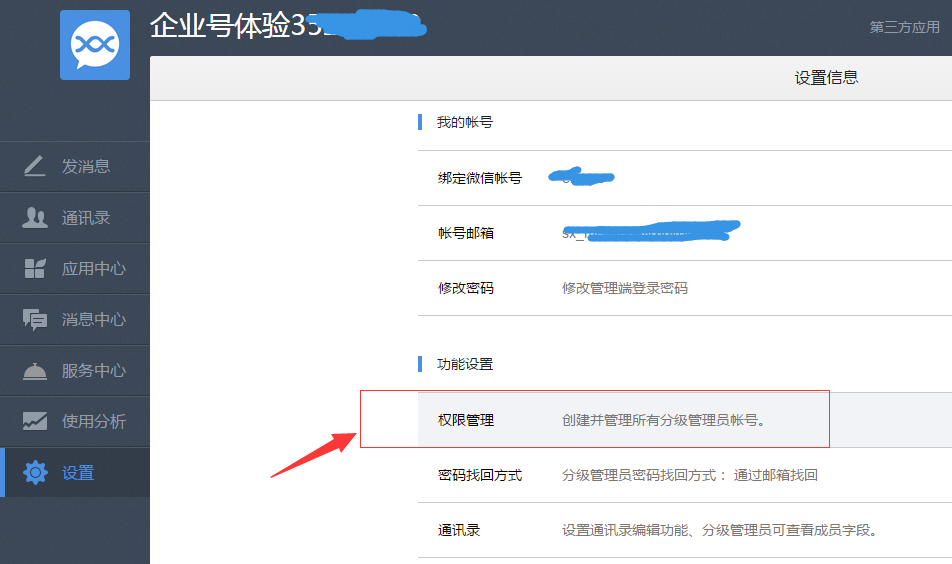
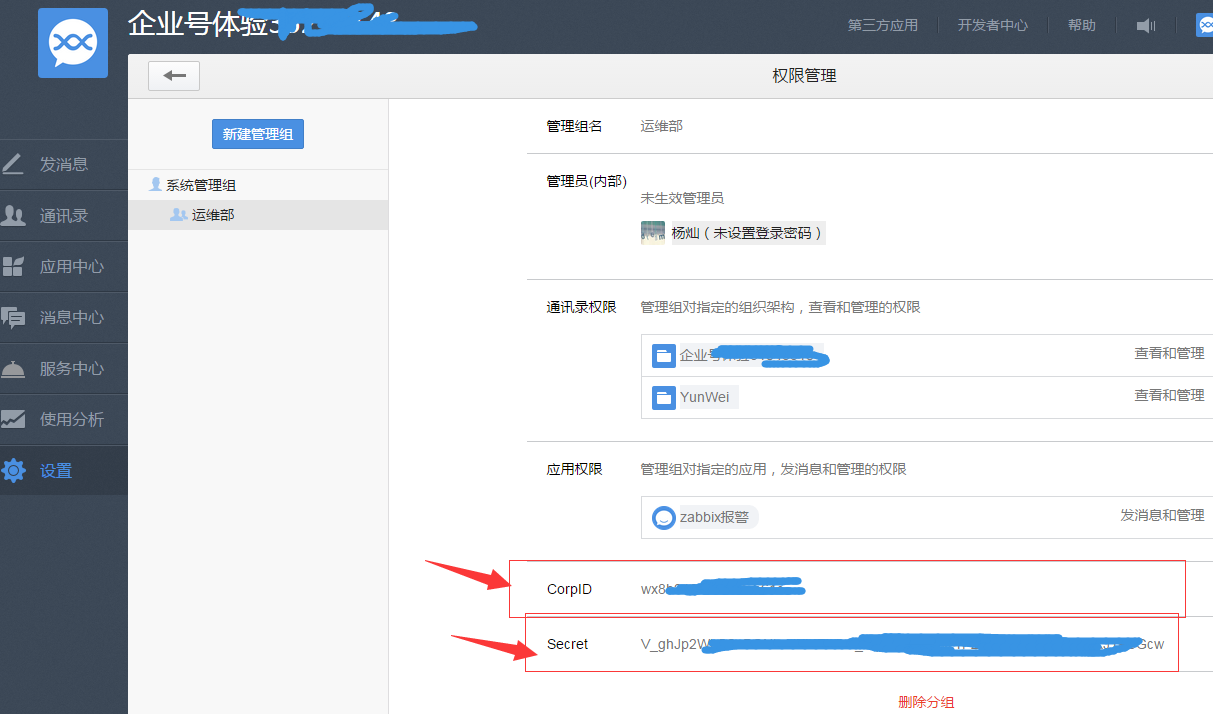
后续工作 TODO
- 增加信息发送频率的限制
这个应用的场景是,在有大量报警的前提下,为了不在短时间范围内一下收到太多短信轰炸,做一个限制多少分钟内发送指定的接收人不超过多少条信息.
这个功能其实已经用shell处理好了,只是python这段代码没有事件修改.